Struggling with Homework on Dijkstra's algorithm or its C++ implementation? Our team of experts is ready to assist you in your CPP Homework. We provide detailed guidance, personalized assistance, and practical resources to help you master this critical tool in computer science. Whether you're a beginner or experienced, we're here to facilitate your learning journey.
- Algorithms are the fundamental building blocks that shape our digital experiences in the vast and dynamic field of computer science. We frequently aren't aware of the underlying complexities that enable us to communicate, travel, shop, and more with ease as we maneuver through a maze of data in our daily lives. Dijkstra's algorithm is one such fundamental tool that operates in the background and enables us to map our paths through this maze of data.
- This algorithm, developed in 1956 by Dutch computer scientist Edsger Dijkstra, has greatly influenced how computer scientists approach problem-solving. Dijkstra's algorithm, which is fundamentally a pathfinding algorithm, is used to determine the shortest path between a given source node and all other nodes in a graph. The idea of this "graph" encompasses a network of nodes connected by lines or "edges," rather than just a graphic representation.
- The Dijkstra algorithm serves as the foundation for many modern technologies on which we rely heavily. Have you ever wondered how your GPS system quickly determines the shortest path to your destination while taking all feasible routes into account? Or how does an Internet Protocol (IP) network guarantee that data packets take the shortest path to their destination? The magician working behind the scenes, orchestrating these tasks with incredible accuracy, is Dijkstra's algorithm.
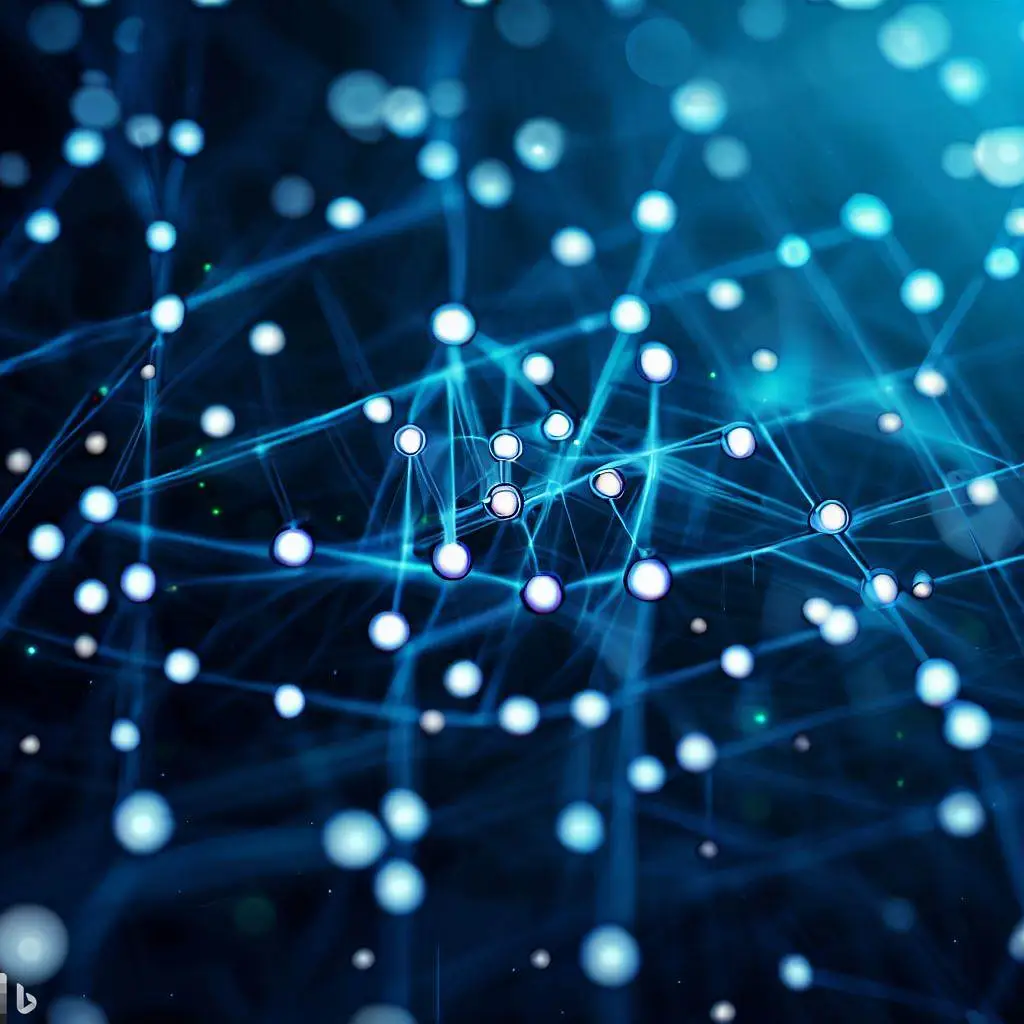
- Despite being widely used, Dijkstra's algorithm is frequently misunderstood or ignored by people who are just entering the field of computer science. The difficulty in comprehending and applying this algorithm, however, is by no means insurmountable. Dijkstra's algorithm can be a manageable and rewarding part of your programming toolkit, particularly when used with a language as flexible and user-friendly as C++.
- In this post, we'll explore the complexities of Dijkstra's algorithm, starting with its fundamental idea and moving on to examples from real-world applications and a detailed C++ implementation. As a result, whether you're an experienced programmer looking for a refresher or a novice programmer eager to advance your skills, this in-depth look at Dijkstra's algorithm will be an invaluable aid in your coding journey.
Dijkstra algorithm:
Dijkstra's algorithm, which is named after the Dutch computer scientist Edsger Dijkstra, is a marvel in the field of pathfinding algorithms. It provides precise solutions for a wide range of issues in computer science and operations research because it is built to find the shortest paths from a source node to all other nodes in a graph. Dijkstra's algorithm is essential for a variety of tasks, including accurately mapping the shortest route on your GPS and effectively routing data packets across computer networks.
The Theoretical Basis:
It's crucial to understand the fundamental idea behind Dijkstra's algorithm before moving on to the implementation. It uses a graph, or network of nodes (or vertices) connected by edges, as its operating system. A weight is attached to each edge, signifying the "cost" of moving from one node to another. These weights can represent a variety of real-world quantities, including time, distance, and even monetary value.
The algorithm starts at the source node and scans the nodes in its immediate vicinity. The node with the lowest weight is chosen, designated as "visited," and the process continues. This procedure is repeated, always selecting the source node that hasn't been visited yet from those with the smallest cumulative weight. The algorithm keeps going until every node has been reached and the shortest path has been found.
Benefits and applications of the Dijkstra algorithm
The Dijkstra algorithm has numerous uses in a variety of fields. In terms of transportation, GPS systems use it to determine the shortest path between points, greatly reducing travel time. By locating the network's fastest path, it aids in the routing of data packets across the internet in telecommunications. For building shortest path algorithms in map-based location services and intelligent agents, it is used in computer science and machine learning. It can identify the most economical delivery or transportation routes in supply chain and logistics.
The Dijkstra algorithm also has a number of benefits. If all weights are positive, it guarantees the shortest path between any two points in a graph. It is effective. It works well with large graphs and is simple to implement in many programming languages. Furthermore, the algorithm can be applied to real-time pathfinding problems because it doesn't require prior knowledge of the entire graph structure or all edge lengths. The algorithm is a mainstay in the field of computer science and beyond due to its adaptability and effectiveness.
Implementing C++: A Deep Dive
Let's move on to the algorithm's actual implementation in C++ now that the theory is clear. Our graph is represented by an adjacency matrix. The weight of the edge connecting nodes 'i' and 'j' is contained in the cell graph[i][j] of this two-dimensional array. We represent the absence of an edge between two nodes with a value of infinity (INT_MAX), which denotes an impassable path.
We use two arrays in addition to the graph: visited[] and distance[]. The shortest distance between each node and the source node is stored in the distance[] array. With the exception of the source node, which is set to 0, all of these distances are initially set to INT_MAX (infinity), since a node's own distance is zero. The boolean array visited[] keeps track of the nodes we have visited, with all nodes starting as 'false'.
The algorithm's main component is a loop that keeps going until all nodes have been visited. The smallest distance node that hasn't been visited inside of this loop is the one we designate as "visited." If the new path to a neighbor is shorter than the previously known shortest path, we iterate over the neighboring nodes of the current node, updating the distance[] values.
Here is a comprehensive example of C++ code that uses Dijkstra's algorithm:
#include
using namespace std;
#define V 5
int minDistance(int dist[], bool visited[]) {
int min = INT_MAX, min_index;
for (int v = 0; v < V; v++)
if (visited[v] == false && dist[v] <= min)
min = dist[v], min_index = v;
return min_index;
}
void dijkstra(int graph[V][V], int src) {
int dist[V];
bool visited[V];
for (int i = 0; i < V; i++)
dist[i] = INT_MAX, visited[i] = false;
dist[src] = 0;
for (int count = 0; count < V - 1; count++) {
int u = minDistance(dist, visited);
visited[u] = true;
for (int v = 0; v < V; v++)
if (!visited[v] && graph[u][v] && dist[u] != INT_MAX && dist[u] + graph[u][v] < dist[v])
dist[v] = dist[u] + graph[u][v];
}
}
The Dijkstra algorithm is fundamentally implemented in this C++ code. It does not, however, deal with more intricate situations like finding the shortest route between two particular nodes, dealing with cycles in the graph, or handling graphs with negative edge weights.
Conclusion:
- Following this journey through Dijkstra's Algorithm, it is obvious that knowing how to use this algorithm and being able to understand it are essential skills in any programmer's toolkit. Despite appearing to be complex, this powerful tool can be easily understood when dissected step by step, as we did with the help of its theory and C++ implementation.
- With its effective and reliable approach to problem-solving, Dijkstra's Algorithm has a wide range of applications, from computer networks to GPS systems. Its key advantage is its speedy computation of shortest paths, which makes it the preferred algorithm for any issue that can be represented as a graph with weighted edges.
- However, it's crucial to keep in mind that no one algorithm can resolve all issues. The Dijkstra algorithm has some drawbacks, like the incapacity to deal with negative edge weights. The secret to using computer science tools effectively is knowing when and how to use each one.
- The journey we've been on today to comprehend and apply Dijkstra's Algorithm in C++ is evidence of how beautifully theory and application in computer science interact. It also serves as a reminder of the vastness, fascination, and potential for improving, streamlining, and connecting our lives in the world of algorithms.
- As we come to a close with this exploration, keep in mind that learning is a continuous process. Every step you take toward mastering the art and science of coding, whether you're an experienced programmer or a beginner, strengthens your foundation. One algorithm at a time, keep experimenting, putting it into practice, and keep improving the digital world.