It can be difficult to get started on a C++ homework assignment because it necessitates a thorough comprehension of the complex concepts in the language. It is crucial to delve deeply into the subject, exposing the layers of knowledge and real-world applications, in order to meet your professor's expectations. With advice on how to show that you fully understand your C++ homework, this blog aims to give you invaluable insights into what your C++ professor really expects of you. It's important to understand that your professor is looking for more than just code that compiles and runs as you explore the world of C++. They are looking for proof that you can comprehend the fundamental ideas, use problem-solving strategies, and show an understanding of how C++ concepts relate to one another. Understanding concepts like object-oriented programming, data structures, memory management, and algorithm design is necessary for language mastery.
It can be difficult to get started on a C++ homework assignment because it necessitates a thorough comprehension of the complex concepts in the language. It is crucial to delve deeply into the subject, exposing the layers of knowledge and real-world applications, to meet your professor's expectations and learn how to complete your C++ homework. With advice on how to show that you fully understand your C++ homework, this blog aims to give you invaluable insights into what your C++ professor expects of you.
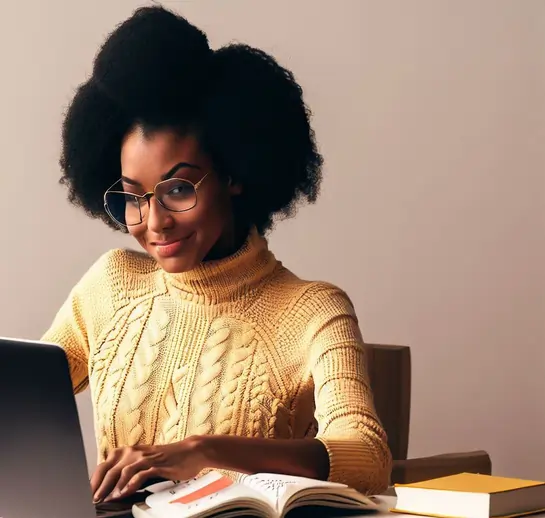
It's important to understand that your professor is looking for more than just code that compiles and runs as you explore the world of C++. They are looking for proof that you can comprehend the fundamental ideas, use problem-solving strategies, and show an understanding of how C++ concepts relate to one another. Understanding concepts like object-oriented programming, data structures, memory management, and algorithm design is necessary for language mastery.
Your professor emphasizes the significance of not just finishing the assignments but also showcasing your understanding of the underlying concepts because she wants to see you develop as a programmer. It's important to carefully read the assignment prompt, taking note of any specific specifications, limitations, and desired results. You can then modify your solution to meet the requirements. Your professor also wants you to show that you know how to write clear, effective, and well-documented code. To effectively communicate your understanding of the codebase, you must use proper coding conventions, meaningful variable names, and clear comments.
Syntax, variables, and Data Types: Understanding the Basics:
The first thing your professor will look for in your C++ homework is that you have a firm grasp of the fundamentals. Programming successfully in C++ requires a solid understanding of syntax, variables, and data types.
C++ is incredibly precise in terms of syntax. Your program could crash as a result of something as simple as an extra parenthesis or a missing semicolon. Your professor will be looking for well-written, mistake-free code that adheres to the proper syntax conventions. This entails correctly declaring variables, applying the appropriate operators, and putting control structures like loops and conditional statements into use, among other things.
Additionally essential to C++ are variables and data types. Data types specify the kinds of information that can be stored in a variable, whereas variables are used to store information. Data types in C++ range from integers to characters to booleans to floating-point numbers and more. It is crucial to comprehend these data types and know when to use them. You must declare and initialize your variables properly, use the right data types for the task at hand, and meet your professor's expectations.
Using Control Structures, Functions, and Modularity to the Fullest:
Control structures are an essential component of all programming languages. Specifically, loops (for, while, do-while) and conditional statements (if, switch) enable your code to make choices and repeat actions. They serve as the foundation for more complex programs.
Your professor will review your C++ homework to ensure the proper application and use of these control structures. This entails employing loops when necessary to prevent repetitive code, correctly implementing conditional statements to direct the flow of your program, and generally displaying a thorough understanding of how these structures operate. The application of these structures should also be logical and aid in the success of the solution.
Next, a key idea your professor will be looking for you to demonstrate is how to use functions correctly and understand how C++'s modularity works. A series of code statements called a function to carry out a particular task. They give your code organization, increase readability, and make it reusable.
The effective use of functions in your C++ homework demonstrates that you are aware of how to "decompose" a problem into smaller, more manageable tasks, which is a crucial skill in software development. Your professor will be looking for clearly defined functions with appropriate input parameters and return types that serve a specific purpose.
Memory Management and Object-Oriented Programming (OOP) in C++:
The focus of the object-oriented programming language C++ is on objects and classes. Your professor will be looking for an understanding of the OOP tenets of encapsulation, inheritance, polymorphism, and abstraction in your C++ homework.
By correctly defining classes, producing objects, and utilizing them in a way that boosts your program's organization and effectiveness, you can demonstrate these principles in your homework. It will also be interesting to look at how inheritance and polymorphism can be used to make code that is more adaptable and extensible. Finally, it will be expected that you will use abstraction and encapsulation to protect your data and restrict the scope of your classes and objects.
Your professor will closely examine any features of C++ that enable low-level memory manipulation, such as pointers. You must be able to use pointers, allocate and deallocate memory, and steer clear of pitfalls like memory leaks if you want to impress your professor.
Debugging and handling errors:
Your ability to debug code and effectively handle errors is yet another crucial component of programming that your professor will be looking for in your C++ homework. This entails adding features to your code that foresee and address potential issues in addition to finding and fixing syntax, logic, and runtime errors. This could include input verification to ward off user mistakes or exception management tools to deal with unforeseen events as they arise.
Your professor expects you to show off your programming knowledge and problem-solving abilities in addition to your command of the C++ language in your homework assignments. Additionally, it is crucial that you can write clear, effective, and error-free code. As you progress through your C++ learning process, keep in mind that repetition and consistency are essential for mastering this potent programming language.
Advanced C++ Concepts: STL and Templates:
Your professor will start to assess your comprehension and application of more complex ideas, like C++ templates and the Standard Template Library (STL), once you have mastered fundamental C++ and OOP concepts.
With the help of templates and a C++ feature, you can make your functions and classes work with different data types without having to write duplicate code. The idea behind generic programming is this. Your professor will anticipate that you can correctly use class and function templates and that you are aware of how they improve the efficiency and reuse of your code.
A C++ library called the Standard Template Library (STL) has several predefined templates, such as collections of algorithms and data structures. Knowledge of the STL library, which enables you to carry out complex operations and more effectively manage data collections, demonstrates a thorough understanding of C++. The proper and effective use of STL elements like vectors, lists, sets, maps, and more will be evaluated by your professor.
Code Optimization and Efficiency in C++:
A crucial component of any programming language, including C++, is code efficiency. Your professor will be expecting you to show that you understand how to write code that not only effectively solves problems but also does so.
This means that you need to pay attention to your code's space complexity (how much memory your code uses) and time complexity (how your code's running time grows as the size of the input increases). The code must employ effective algorithms that can handle large amounts of data without consuming an excessive amount of resources or taking an excessive amount of time to execute.
The performance of your code can also be enhanced by optimization strategies like using effective data structures or reducing the number of computations. Remember that writing efficient code involves more than just making it run more quickly. It involves making sure your code works effectively and consistently regardless of the volume or complexity of the input.
Conclusion:
In conclusion, doing your C++ homework according to your professor's expectations involves more than just finishing the current tasks. It entails delving deeply into the material, comprehending the underlying ideas, and using problem-solving methods to produce effective and tasteful solutions.
You can demonstrate a thorough understanding of your C++ homework by thoroughly understanding the assignment's instructions, realizing how closely related the various C++ concepts are, and concentrating on writing clean, well-documented code. Use the tools your professor has provided, such as textbooks, online tutorials, and additional reference materials, to advance your understanding and sharpen your programming abilities.
Keep in mind that learning C++ is a journey that calls for constant exploration and practice. Accept challenges, ask for help when you need it, and work to get better at understanding each assignment. Your professor's expectations are made to challenge you to the limit and make sure you gain a firm grounding in C++ programming.
Consider C++ homework assignments as valuable opportunities to show off your skills, confirm your knowledge, and prepare for future success in the field as you move forward in your academic career. You will undoubtedly meet and surpass your professor's expectations if you remain curious, persistent, and committed to developing your skills.